In-App Purchases
Reminder - Keep Daily Backups
When working on a strategy game with a kit as big as the Complete Kit - always keep a working daily backup! Save yourself the trouble of rolling-back changes and losing work.
What are in-app purchases?
In-App Purchases allow your game players to spend real currency to get in-game resources such as virtual rare currency (gems, for example) or filling their resources (gold, for example). The Game Kit is setup to support In-App Purchases using either Unity IAP or your own IAP asset.
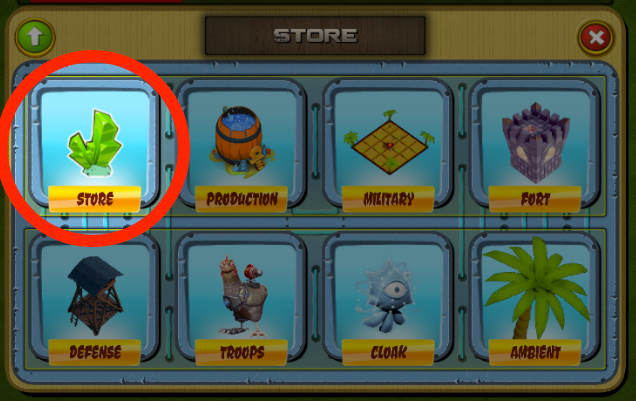
Click to view larger.
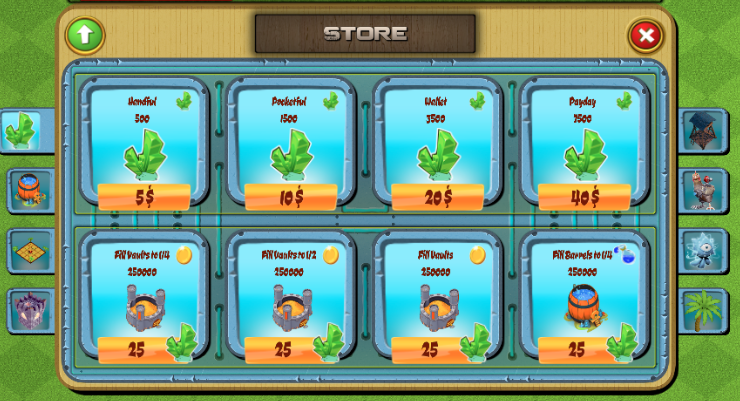
Click to view larger.
You can choose whatever currency type you want to use for your game. The demo uses two - gold and liquid mana plus one rare currency (gems), however these names, images, and all settings can be changed.
For example, some developers may want to add additional currencies in their game such as wood and stone. This can be done by duplicating existing resource references. (Make sure to keep backups!)
Where to find this IAP store menu in Unity?
Open the game scene hierarchy and locate the ScrollView in Canvas > ModalsWindows > ShopWindow > ShopGroup > StorePanel > BottomGroup > Content > Content. Look at the below screenshot.
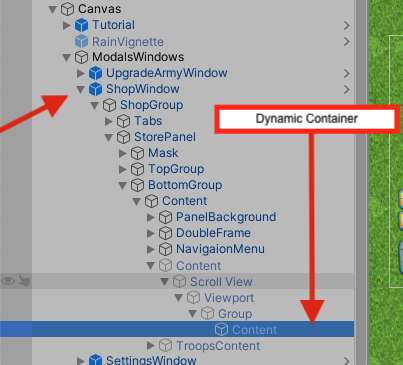
UIAnchor. Click to view larger.
How can players access the In-App Purchase menu?
Either through the shop menu button as seen in the screenshots above, or by clicking the (+) button located next to the crystal stats.
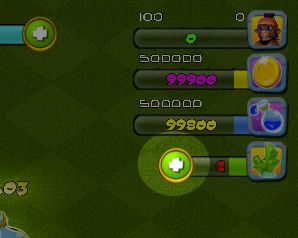
Crystal IAP screen access button
What can you sell in your game?
The Complete Strategy Kit comes with a demo of different purchasable crystal item sets. Using these crystals, you can purchase all the special functions in the gameplay like extra in-game resources or finishing construction or troop training earlier.
Hard Currency (Crystal Gems) | Game starts with 5 free crystal gems. (Can edit this number in GameManager > Stats Inspector) Must use real world currency to buy. Also awarded in random amounts (1-3 crystals) when destroying removable terrain to expand your base. See Terrain > Player Removable Objects documentation for details about the only free way gems are awarded in your game. |
Soft Currency #1 (Gold) | Default is Gold. The first of two currencies generated in the game. You can change this to whatever you wish. |
Soft Currency #2 (Mana) | Default is Liquid Mana. The second of two currencies generated in the game. You can change this to whatever you wish. |
Builders (called Dobbits) | Default is 1. To create two buildings at once, at least 2 builders are required. See Shop Menu > Extra Builders documentaiton for details |
You could technically sell more, as the full source code is included so developers can edit the game to sell any sort of additional bonuses or sets. What we'll talk about in the rest of this documentation page is what's already included, and prescripted for you.
Rare Gem In-App Purchases ScriptableObject (StoreCategory.asset)
Inside Assets/CityBuildingKit/Resources/Assets/StoreCategory.asset you'll find the text behind the IAP store menu. To customize any item in the menu, you simply change the values in this SO(ScriptableObject) file, save, and run the Game scene.
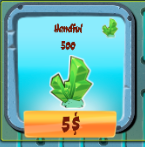
XML Example image
For the above example image of a Handful of gems seen in the kit's UI, below is the SO behind this seen in StoreCategory.asset:
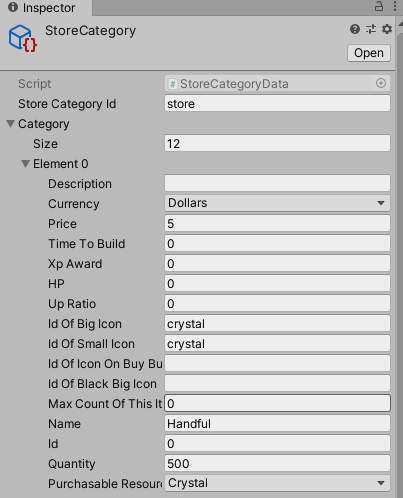
As you can see above, customizing these values is simple. Specify the name, a quantity, the price of your rare currency, etc...
IAP Store Menu Initization
The Scripts/Menus/Shop/ShopController.cs controls everything in the Shop menu. For example, the above data from the SO file is loaded with OnStoreCategoryHandler()
public void OnStoreCategoryHandler(int categoryIndex)
{
OnOpenShop();
IEnumerable<DrawCategoryData> drawCategoryData;
object category;
switch (categoryIndex)
{
case 0:
category = ShopData.Instance.StoreCategoryData;
drawCategoryData = ((StoreCategoryData) category).Category.Select(item =>
new DrawCategoryData {BaseItemData = item, Id = item, Name = item});
DrawCategory(drawCategoryData, ShopCategoryType.Store);
break;
. . .
After the SO data is loaded from - the interface is updated with InitUIStoreItems() (Store.cs) that sets each of the rare currency items for sale in the menu with the data from SO.
public void InitUIStoreItems()
{
UpdateResources();
UpdateUI(6, Stats.Instance.maxGold, existingGold, 4, false);
UpdateUI(7, Stats.Instance.maxGold, existingGold, 2, false);
UpdateUI(8, Stats.Instance.maxGold, existingGold, 1, false);
UpdateUI(9, Stats.Instance.maxMana, existingMana, 4, false);
UpdateUI(10, Stats.Instance.maxMana, existingMana, 2, false);
UpdateUI(11, Stats.Instance.maxMana, existingMana, 1, false);
}
Where to integrate your in-app purchase solution:
By default, the kit is setup to initiate purchases (menu and scripting provided). Purchases are delivered instantaneously for testing. The following excerpt from Scripts/Menus/Shop/Store.cs. shows where you can initiate your IAP scripting:
// Please see:
// https://bitbucket.org/Unity-Technologies/unity-iap-samples
//
public void BuyStoreItem(DrawCategoryData data)
{
var itemData = data.BaseItemData as StoreCategory;
if (itemData == null)
{
return;
}
switch (itemData.purchasableResource)
{
case GameResourceType.Crystal:
BuyCrystal(itemData);
break;
case GameResourceType.Gold:
if (itemData.GetId() == 6)
{
goldSelection = 0;
}
else if (itemData.GetId() == 7)
{
goldSelection = 1;
}
else if (itemData.GetId() == 8)
{
goldSelection = 2;
}
UpdateResources ();
BuyGold(itemData);
break;
case GameResourceType.Mana:
if (itemData.GetId() == 9)
{
manaSelection = 0;
}
else if (itemData.GetId() == 10)
{
manaSelection = 1;
}
else if (itemData.GetId() == 11)
{
manaSelection = 2;
}
UpdateResources ();
BuyMana(itemData);
break;
}
Stats.Instance.UpdateUI();
Stats.Instance.UpdateCreatorMenus();
}
In-Game Resource Filling
In addition to purchasing rare currencies like gems with real currency - you can offer the option for game users to fill their resources with gems. These are not paid with real currency but instead gems so you can change the values dynamically.
Here's a screenshot from the IAP store menu to fill in-game currency #1 (gold, in this case) by 25%, 50%, and 100%.
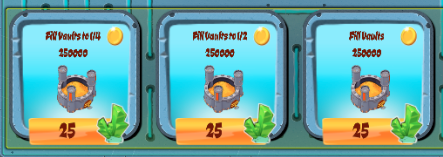
IAP to fill player resources
to
Unity In-App Purchase Sample Code
For actually processing real money transactions you'll need an Apple Developer account or Google Publisher account. Then, use Unity's built-in IAP solution for connecting and processing transactions. Reference code and documentation is available here: https://bitbucket.org/Unity-Technologies/unity-iap-samples
Initiate the purchase in the functions listed above.
Video: Unity In-App Purchasing Demonstration
Other Recommended IAP Assets?
If you're looking to integrate IAP into your game, we recommend using the Unity IAP demo source code mentioned above (available here) -- but otherwise if you need an alternative - the Simple IAP Unity asset available for download from the Unity Asset Store is a good second choice.
Where can I edit the crystal price for unit training time?
The following excerpt from Scripts/Units/MenuUnit.cs shows the game settings for how the total price is computed in crystals based on the total minutes remaining in the production queue.
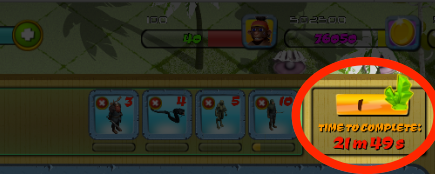
Unit crystal finish now cost. Click to view closer.
For example, if time remaining is less than 30 minutes then it only costs one crystal gem to finish production of all units. Once the total time remaining exceeds 30 minutes but is less than 60 minutes the price increases to 3 crystal gems, and so on.
You can adjust these settings in MenuUnit.cs. Look for the following source code:
if (timeRemaining >= 4320) priceInCrystals = 150;
else if (timeRemaining >= 2880) priceInCrystals = 70;
else if (timeRemaining >= 1440) priceInCrystals = 45;
else if (timeRemaining >= 600) priceInCrystals = 30;
else if (timeRemaining >= 180) priceInCrystals = 15;
else if (timeRemaining >= 60) priceInCrystals = 7;
else if (timeRemaining >= 30) priceInCrystals = 3;
else if (timeRemaining >= 0) priceInCrystals = 1;
Where can I edit the crystal price for building construction time?
Like the unit training excerpt above, another way to use crystal gems is to accelerate construction times rather than wait the time remaining. Pressing the crystal button finishes the construction instantly.
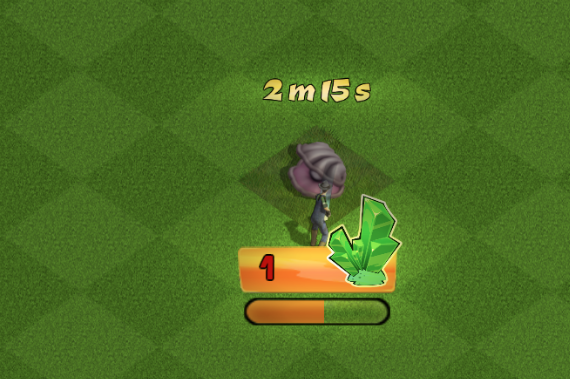
Building crystal finish now cost. Click to view closer.
Look at the Scripts/Creators/ConstructionSelector.cs UpdatePrice function which updates the crystal price label on construction projects.
// Scripts/Creators/ConstructionSelector.cs
//update the price label on the button, based on remaining time
private void UpdatePrice(int remainingTime)
{
/*
Summary of Gem Prices
Minute Range Cost in Gems
0 - 30 1
30 - 60 3
60 - 180 7
180 - 600 15
600 - 1440 30
1440- 2880 45
2880- 4320 70
4320+ 150
*/
if (remainingTime >= 4320) { priceno = 150; }
else if (remainingTime >= 2880) { priceno = 70; }
else if (remainingTime >= 1440) { priceno = 45; }
else if (remainingTime >= 600) { priceno = 30; }
else if (remainingTime >= 180) { priceno = 15; }
else if (remainingTime >= 60) { priceno = 7; }
else if (remainingTime >= 30) { priceno = 3; }
else if(remainingTime >= 0) { priceno = 1; }
((tk2dTextMesh)Price.GetComponent("tk2dTextMesh")).text = priceno.ToString();
}
Can I also sell ad unlocks as an In-App Purchase?
Yes, you can sell pay for an ad-free game experience options. A good example to look at is the fill resource options in the In-App Purchase store and the Store.cs script.
The easiest way to do this is to setup ads in your application that display only if a new Stats variable like AdFree boolean is set to false.
Add the Pay to Unlock item into the In-App Purchase store menu with a crystal price like how the refill resources use crystals to change the resource stats. Then when the button is triggered, deduct the crystal currency and update the Stats AdFree boolean to true. The Stats are carried between save and loads so your players will never see the ads again.
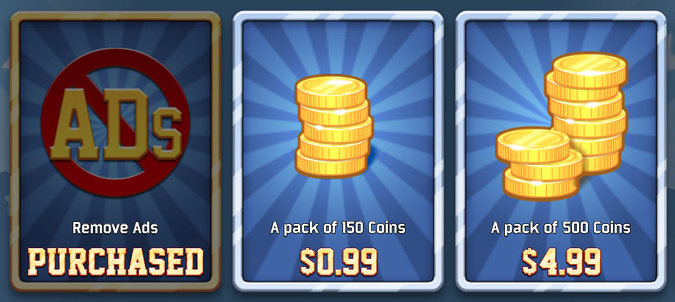
Screenshot example from other iPhone games
Before starting to add new features like this - please get comfortable with the scripting first. Read the functions in the Store.cs script and use Ctrl+F in the Scripts folder to locate how functions work between scripts. The kit is a huge package of interconnected features and scripts, so it's better to get comfortable first with the scripting before adding new features that might affect other gameplay elements.
Updated less than a minute ago