Campaign
Reminder - Keep Daily Backups
When working on a strategy game with a kit as big as the Complete Kit - always keep a working daily backup! Save yourself the trouble of rolling-back changes and losing work.
What is campaign?
Campaign allows players to battle scenarios of increasing difficulty with maps you've premade. Attacking players gain resources and enter battle just like as if it's against a normal player, however they pick the type of base they want to attack in the Army menu by selecting one of the campaign map locations.
These campaign locations aren't technically enemy players - so although the attacking player can destroy these bases and gain loot, the bases don't decrease in value. They remain with the same value for each player as you would expect campaign maps to perform.
We've designed 21 map buttons in the kit but you can customize this however you wish. The full source code is included. For example, you can edit these maps regularly on your server to provide monthly fresh content. Or make extremely difficult challenges for players.
The choice is yours. Developers that don't want campaign elements in their game can remove the campaign options from the army menu (Game scene - Anchor center: army). Removing these elements will automatically disables campaign from your game.
If you want to use the campaign element in your game, there's lots of variations you could try. Here are a few ideas to start that don't require a lot of changes:
Campaign Variation Ideas | Description |
---|---|
Campaign Villages | Let the player battle one of the 21 campaign maps. No changes necessary. |
Daily Challenge Maps | Let players challenge the Map-of-the-day from 31 that you choose. |
Level Up Villages | Increasing difficulty, depending on whether the user can defeat the previous level. |
Top Player Villages | Instead of delivering maps you designed, feature your top players. Upload like the daily challenge. |
Sandbox Villages | Use the single-player campaign as a sandbox to let users try out troops on a sample map. |
Campaign Menu
When going to battle, the player can select any one of the campaign buttons to battle premade campaign maps or the pick a fight to battle players. The campaign menu is a scroll view you can click and drag to see more. Below is a screenshot of the layout and background images you can customize in the Game scene, Anchor Center, army element.
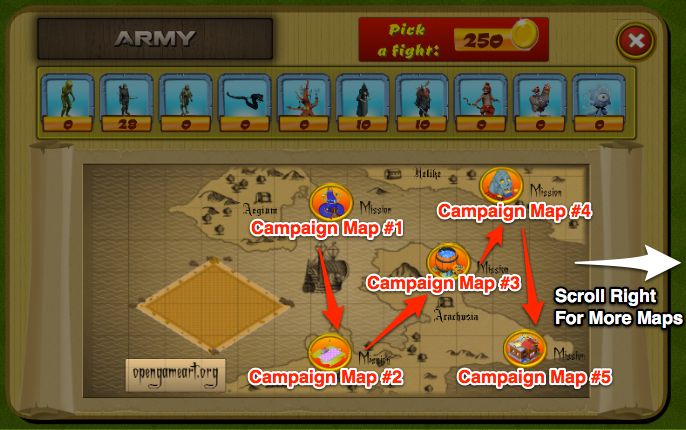
Click to view closer.
We've included a scroll box so you can let players scroll through all of the campaign map options. By default, we've enabled them all for developers to test - although if you would like to progressively unlock campaign levels then you'll want to add this as a variable that is generated on the battle complete menu in Helios.cs, transfered with TransData.cs and stored as a new setting using SaveLoadMap.cs.
A good example to look at is the ((TransData)transData).tutorialBattleSeen variable which tracks if the user has seen the battle tutorial and stores that permanently with their player base as ((StatsBattle)statsBattle).tutorialBattleSeen.
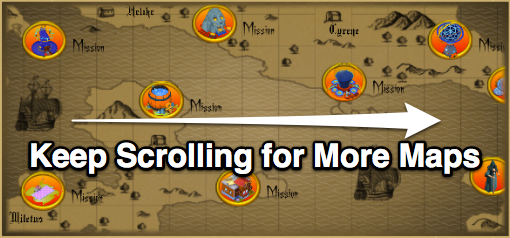
Click and drag the menu to view more campaign maps. Everything is customizable.
What script is involved?
When the user goes to battle (the Game scene Anchor Center - army menu) they can either select a random player base to battle or pick one of the campaign maps in the campaign scrollview. The Scripts/Menus/Army/MenuArmy.cs controls everything that happens on the army menu.
If they pick one of the 21 campaign buttons, one of the LoadCampaign() function associated with those buttons is triggered which sets the campaignLevel variable and runs LoadMultiplayer0() which checks if they have enough units and the campaignLevel variable is set.
The following excerpt from MenuArmy.cs shows you these functions:
// MenuArmy.cs
// Called by the buttons to load the specific campaign level
private void LoadCampaign(int campaignLevel)
{
// Sets the transfer data for battle
// Used by Scripts/Multiplayer/TransData.cs and Scripts/Save/Helios.cs
((TransData)transData).campaignLevel = campaignLevel;
// Start the game loading
LoadMultiplayer0 ();
}
// This function goes through a few error cases that might prevent
// a battle from starting. Such as:
// no units for battle
// no gold (requires 250 to search unless a campaign map)
public void LoadMultiplayer0()
{
bool unitsExist = false;
// Check unit total
for (int i = 0; i < ((Stats)stats).existingUnits.Length; i++)
{
if(((Stats)stats).existingUnits[i]>0)
{
unitsExist = true;
break;
}
}
// If units exist and a campaign level number too - load a campaign map (no charge)
if (unitsExist && ((TransData)transData).campaignLevel != -1) {
StartCoroutine (LoadMultiplayerMap (0));
}
else if(!unitsExist)
{
// No units exist, show an error message
((Messenger)statusMsg).DisplayMessage("Train units for battle.");
}
else if(unitsExist &&
((((Stats)stats).gold >= 250&&((TransData)transData).campaignLevel==-1)))
{
// A normal multiplayer match is starting, go to battle if they have gold and it's not a campaign button press
StartCoroutine(LoadMultiplayerMap(0));
}
else if(!unitsExist)
{
// No units exist, show an error
((Messenger)statusMsg).DisplayMessage("Train units for battle.");
}
else
{
// No gold - show an error
((Messenger)statusMsg).DisplayMessage("You need more gold.");
}
}
If the player has units and clicked a campaign button, the above reference code starts the coroutine LoadMultiplayerMap(0) which basically sets the transfer data, loads the battle scene (Map01 scene) and calls Scripts/Save/SaveLoadBattle.cs to take over with loading the enemy base.
The following excerpt from SaveLoadBattle.cs shows the DownloadBattleMap() function which connects to the server and requests either a random player map or in this case since the campaignLevel variable was carried over from the transfer data - it requests one of the 21 campaign maps (0000camp00 - 0000camp20) as seen in the below excerpt:
// Excerpt from SaveLoadBattle.cs
// Second step after the transdata move from
// the player base to the battle map
//
IEnumerator DownloadBattleMap()
{
// Get the player's ID for telling the server what map is the player's map
// This prevents accidentally downloading the player's map as an enemy base
// (loads a map other than the user map)
if(CheckServerSaveFile())
ReadMyMapID();
else
myMapIDCode = nullMapIDCode;
// Get the campaign level from the transfer data
// If not set, this means it's a player battle requested
// and not a campaign battle requested
int campaignLevel = ((TransData)transData).campaignLevel;
// Normal player versus player battle
// Requests a random player map that doens't match the player's map ID
// This connects to the server address and file address
// Setup in the Map01 scene GameManager > SaveLoadBattle Inspector
//
if (campaignLevel == -1)
w2 = new WWW (serverAddress + filesAddress + "?get_random_map=1&mapid=" + myMapIDCode + "&license=" + license); //mapid with the get_random_map to prevent the user's map from being downloaded by accident
else
{
// A campaign map has been requested
// Sets the battleMapID from the 21 available (0000camp00 - 0000camp20)
battleMapID = "0000camp" + campaignNo [campaignLevel];
// Requets the battle map contents from the server scripts
// If you don't have these server scripts yet -- login to
// CityBuildingKit.com/download to get them or contact us for help
w2 = new WWW (serverAddress + matchAddress + "?get_user_map=1&mapid=" + battleMapID + "&license=" + license);
}
// Returns the map data
yield return w2;
// ..... to learn more about the rest of this loading process
// ..... see the Finding Battles documentation page
Once the map data is returned, DownloadBattleMap continues to verify the data and then loads the map by triggering the function LoadGame()
Where are campaign maps stored?
Read the Online Server Sync > Admin Player Management documentation page for more details about how you can view and edit campaign maps using the server scripts included with the City building Kit. If you don't yet have these server scripts, please download them from CityBuildingKit.com/download or contact us for help.
How to test campaign?
To test campaign, make sure you've added your license code to Map01 scene GameManager > SaveLoadBattle. Play the Unity scene, train 10-20 army units and then open the Army menu to view the campaign maps. Click any of the campaign buttons to go to battle against the bases.
Can I see some example maps?
We've included examples below, but you can get the full set 0000camp00 - 0000camp20 in the following download link:
v7.2 Sample Maps Download Link
https://www.dropbox.com/s/5clocy6v6v5f0f2/v7_demo_map_files.zip?dl=0
Campaign Map #1
We've included a few image previews of the campaign maps included with the server scripts. The following example is the first campaign map, a simple base with one cannon and two resource storage barrels. This map is 0000camp00.txt included in the server map files (download link above).
Each of these maps are samples you can change - read details further below at the bottom of this page for how to create your campaign maps using the City Building Kit.
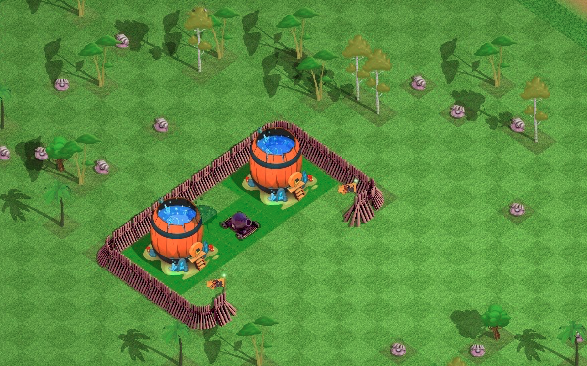
Click to view closer.
The source code for the 1st sample campaign map (0000camp00.txt) is included below:
###StartofFile###
###PosStruct###
Weapon,Cannon,118,2,-384,362
Building,Barrel,119,3,-128,633.5
Building,Barrel,120,3,-768,181
###GridStruct###
WoodFence,WoodFenceNE,93,22,15
WoodFence,WoodFenceNE,94,22,16
WoodFence,WoodFenceNE,95,22,17
WoodFence,WoodFenceNE,96,22,18
WoodFence,WoodFenceNE,97,22,19
WoodFence,WoodFenceNE,98,22,20
WoodFence,WoodFenceNE,99,22,21
WoodFence,WoodFenceNE,100,22,22
WoodFence,WoodCornerS,101,17,14
WoodFence,WoodCornerW,102,22,14
WoodFence,WoodFenceNW,103,21,14
WoodFence,WoodFenceNW,104,20,14
WoodFence,WoodFenceNW,105,19,14
WoodFence,WoodFenceNW,106,18,14
WoodFence,WoodCornerN,107,22,23
WoodFence,WoodFenceNW,110,21,23
WoodFence,WoodFenceNW,111,20,23
WoodFence,WoodFenceNW,112,19,23
WoodFence,WoodFenceNW,113,18,23
WoodFence,WoodCornerE,115,17,23
WoodFence,WoodEndSW,116,17,22
WoodFence,WoodEndNE,117,17,15
###Construction###
###Removables###
TreeA,0,0,2,30
ClamA,2,0,3,4
ClamA,3,0,3,6
TreeA,4,0,3,7
TreeD,5,0,3,31
TreeC,6,0,4,12
ClamA,7,0,4,23
ClamA,8,0,4,27
ClamB,9,0,5,22
TreeD,10,0,5,30
TreeB,11,0,6,9
TreeB,12,0,6,22
TreeA,13,0,6,33
TreeC,14,0,7,17
ClamC,15,0,7,18
ClamC,16,0,7,23
TreeD,17,0,8,12
TreeB,18,0,8,17
ClamA,19,0,8,19
ClamB,20,0,8,32
TreeB,21,0,8,33
TreeA,22,0,9,6
ClamA,23,0,9,18
ClamB,24,0,9,20
TreeD,25,0,9,21
ClamA,26,0,9,31
ClamA,27,0,10,5
TreeB,28,0,10,7
TreeB,29,0,10,12
TreeA,30,0,11,9
ClamA,31,0,11,18
TreeD,32,0,11,30
TreeB,33,0,11,33
TreeC,34,0,13,5
ClamC,35,0,13,14
ClamA,36,0,13,26
ClamA,37,0,14,7
TreeA,38,0,14,14
TreeA,39,0,15,8
TreeC,40,0,15,29
TreeB,41,0,16,12
ClamC,43,0,16,29
TreeD,44,0,17,5
ClamC,46,0,18,3
ClamA,48,0,18,28
TreeA,49,0,18,30
TreeD,50,0,19,6
TreeC,53,0,20,26
ClamC,54,0,20,28
TreeA,55,0,21,5
TreeC,57,0,21,26
ClamB,58,0,22,3
TreeA,59,0,22,25
TreeB,60,0,23,32
TreeB,61,0,24,14
TreeA,62,0,24,26
TreeB,63,0,25,5
TreeA,64,0,25,16
ClamC,65,0,25,17
ClamC,66,0,25,20
TreeC,67,0,26,8
TreeD,68,0,26,9
TreeD,69,0,26,10
TreeD,70,0,26,16
TreeC,71,0,26,23
TreeA,72,0,26,24
TreeC,73,0,27,5
TreeA,74,0,27,9
ClamA,75,0,27,16
TreeB,76,0,27,31
TreeC,77,0,28,9
ClamB,78,0,28,13
ClamC,79,0,28,15
TreeA,80,0,28,20
ClamA,81,0,28,25
TreeC,82,0,29,14
TreeB,83,0,29,15
TreeB,84,0,29,16
ClamB,85,0,29,19
ClamC,86,0,29,22
TreeA,87,0,29,33
TreeA,88,0,31,22
TreeC,89,0,31,29
ClamB,90,0,32,31
ClamB,91,0,33,6
ClamC,92,0,33,16
###RemovableTimers###
###Numerics###
120,117,118
0.00,0
0,0,0,0,0,0,0,0,0,0
0,0,0,0,0,0,0,0,0,0
0,0,0,0,0,0,0,0,0,0
###Stats###
120,342,1,0,0,100,496400,499900,6696,500000,505000,7502,True,True,True,True,True
11/10/2016 9:35:58 PM
###EndofFile###
Campaign Map #3 Daytime
A preview of the 3rd campaign map included with the server scripts (0000camp02.txt) day and night cycles.
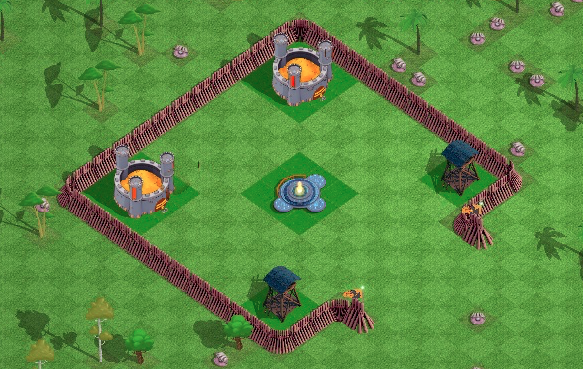
Click to view closer.
Campaign Map #3 Nighttime
The following is a nighttime view. The same day/night cycle scripting runs for the battle scene as it does for the base scene. So the enemy bases could end up in a night cycle depending on how you change the original day night cycle code.
See the Gameplay > Daytime Nighttime Cycles documentation for more details about day / night cycles.
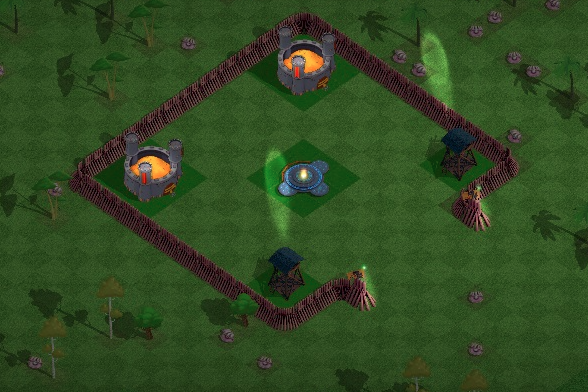
Nighttime view. Click to view closer.
The source code for the 3rd campaign map (0000camp02.txt) is included below:
###StartofFile###
###PosStruct###
Weapon,ArcherTower,146,2,128,-724
Weapon,ArcherTower,147,2,1408,181
Weapon,ArcherTower,148,2,-1280,271.5
Weapon,ArcherTower,149,2,0,1176.5
Building,Forge,150,3,-128,-452.5
Building,Forge,151,3,-512,-181
Building,Vault,152,3,-896,90.5
Building,Generator,153,3,1024,362
Building,Generator,154,3,640,633.5
Building,Barrel,155,3,256,905
Building,Summon,156,3,-768,543
Building,Toolhouse,157,2,-256,995.5
Weapon,Cannon,158,2,768,-271.5
###GridStruct###
WoodFence,WoodFenceNE,98,24,15
WoodFence,WoodFenceNE,99,24,18
WoodFence,WoodFenceNE,100,24,16
WoodFence,WoodFenceNE,101,24,17
WoodFence,WoodFenceNE,102,24,14
WoodFence,WoodFenceNE,103,24,19
WoodFence,WoodFenceNE,104,24,20
WoodFence,WoodFenceNE,105,24,21
WoodFence,WoodFenceNE,106,24,22
WoodFence,WoodFenceNE,107,24,23
WoodFence,WoodFenceNW,108,23,26
WoodFence,WoodFenceNW,109,22,26
WoodFence,WoodFenceNW,110,21,26
WoodFence,WoodFenceNW,111,20,26
WoodFence,WoodFenceNW,112,19,26
WoodFence,WoodFenceNW,113,18,26
WoodFence,WoodFenceNW,114,17,26
WoodFence,WoodFenceNW,115,16,26
WoodFence,WoodFenceNE,116,24,24
WoodFence,WoodFenceNE,117,24,25
WoodFence,WoodCornerN,118,24,26
WoodFence,WoodCornerW,119,24,13
WoodFence,WoodFenceNW,120,23,13
WoodFence,WoodFenceNW,121,22,13
WoodFence,WoodFenceNW,122,21,13
WoodFence,WoodFenceNW,123,20,13
WoodFence,WoodFenceNW,124,19,13
WoodFence,WoodFenceNW,125,18,13
WoodFence,WoodFenceNW,126,17,13
WoodFence,WoodFenceNW,127,16,13
WoodFence,WoodFenceNW,128,15,26
WoodFence,WoodFenceNW,129,14,26
WoodFence,WoodFenceNW,130,13,26
WoodFence,WoodFenceNW,131,12,26
WoodFence,WoodFenceNW,132,11,26
WoodFence,WoodFenceNW,133,15,13
WoodFence,WoodFenceNW,134,14,13
WoodFence,WoodFenceNW,135,13,13
WoodFence,WoodFenceNW,136,12,13
WoodFence,WoodFenceNW,137,11,13
WoodFence,WoodCornerS,138,10,13
WoodFence,WoodCornerE,139,10,26
WoodFence,WoodFenceNE,140,10,25
WoodFence,WoodFenceNE,141,10,24
WoodFence,WoodEndSW,142,10,23
WoodFence,WoodFenceNE,143,10,14
WoodFence,WoodFenceNE,144,10,15
WoodFence,WoodEndNE,145,10,16
###Construction###
###Removables###
ClamA,0,0,2,6
ClamB,1,0,2,13
ClamB,2,0,2,18
ClamA,3,0,2,22
ClamA,4,0,2,27
TreeA,5,0,3,13
TreeD,6,0,3,19
ClamB,7,0,4,7
ClamC,8,0,4,15
ClamB,9,0,5,33
ClamB,10,0,6,17
ClamC,11,0,6,20
TreeB,12,0,6,22
TreeA,13,0,7,17
TreeC,14,0,7,27
TreeC,15,0,8,11
ClamA,16,0,8,17
ClamC,17,0,9,13
ClamB,18,0,9,18
ClamB,19,0,9,21
ClamC,20,0,9,26
ClamB,21,0,9,28
TreeD,24,0,10,33
TreeB,25,0,11,4
TreeB,27,0,11,29
TreeB,28,0,12,8
TreeC,30,0,13,30
TreeC,31,0,13,31
TreeD,33,0,14,27
TreeC,34,0,15,2
ClamB,35,0,15,4
TreeC,36,0,15,8
TreeA,38,0,15,33
TreeD,39,0,16,5
TreeC,40,0,17,3
TreeA,41,0,17,11
ClamB,43,0,18,3
TreeC,44,0,18,6
TreeD,45,0,18,8
ClamB,50,0,20,28
TreeC,54,0,23,4
ClamC,55,0,23,11
ClamC,57,0,23,32
TreeD,58,0,24,2
TreeA,59,0,24,9
ClamC,61,0,25,22
ClamC,62,0,26,3
TreeD,63,0,26,9
ClamB,64,0,26,10
ClamB,65,0,26,23
ClamA,66,0,26,24
TreeA,67,0,26,27
ClamB,68,0,26,33
ClamC,69,0,27,6
TreeB,70,0,28,8
TreeB,71,0,28,16
ClamA,72,0,28,17
ClamA,73,0,29,6
TreeD,74,0,29,15
TreeC,75,0,29,18
ClamB,76,0,29,26
TreeC,77,0,29,28
ClamC,78,0,29,31
ClamC,79,0,30,9
ClamC,80,0,30,13
ClamC,81,0,30,15
TreeB,82,0,30,22
ClamA,83,0,30,23
ClamC,84,0,30,27
ClamA,85,0,31,3
ClamA,86,0,31,16
ClamA,87,0,31,23
TreeB,88,0,31,27
TreeD,89,0,31,28
ClamB,90,0,32,10
TreeD,91,0,32,28
ClamA,92,0,32,31
TreeA,93,0,33,3
TreeD,94,0,33,7
TreeA,95,0,33,20
TreeA,96,0,33,23
TreeB,97,0,33,32
###RemovableTimers###
###Numerics###
157,145,158
0.00,0
0,0,0,0,0,0,0,0,0,0
0,0,0,0,0,0,0,0,0,0
0,0,0,0,0,0,0,0,0,0
###Stats###
158,1489,2,0,0,100,92700,96200,473,502500,502500,500,True,True,True,True,True
11/10/2016 9:56:57 PM
###EndofFile###
Campaign Map #4 Daytime
Here is a screenshot of the the 4th campaign map included with the kit demo (0000camp03.txt)
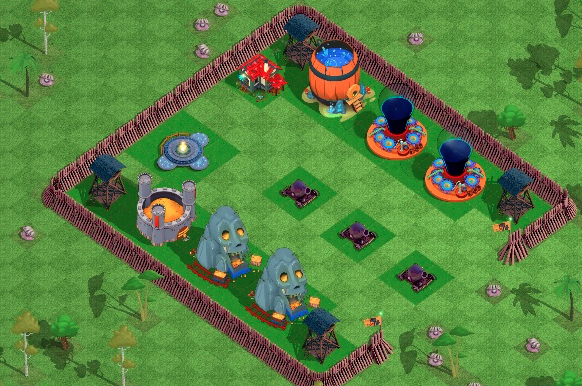
Click to view closer.
Campaign Map #4 Nighttime
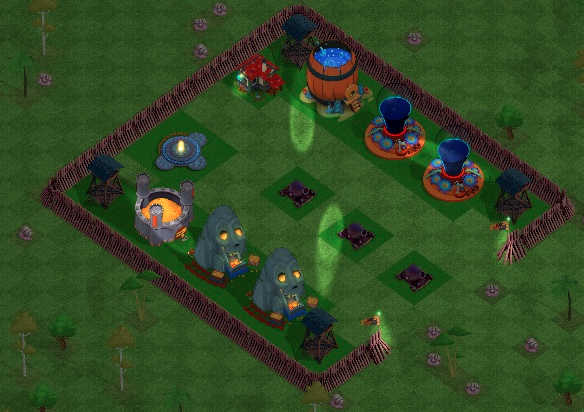
Nighttime view. Click to view closer.
The source code for the 4th campaign map (0000camp03.txt) is included below:
###StartofFile###
###PosStruct###
Weapon,ArcherTower,146,2,128,-724
Weapon,ArcherTower,147,2,1408,181
Weapon,ArcherTower,148,2,-1280,271.5
Weapon,ArcherTower,149,2,0,1176.5
Building,Forge,150,3,-128,-452.5
Building,Forge,151,3,-512,-181
Building,Vault,152,3,-896,90.5
Building,Generator,153,3,1024,362
Building,Generator,154,3,640,633.5
Building,Barrel,155,3,256,905
Building,Summon,156,3,-768,543
Building,Toolhouse,157,2,-256,995.5
Weapon,Cannon,158,2,768,-271.5
Weapon,Cannon,159,2,384,0
Weapon,Cannon,160,2,0,271.5
###GridStruct###
WoodFence,WoodFenceNE,98,24,15
WoodFence,WoodFenceNE,99,24,18
WoodFence,WoodFenceNE,100,24,16
WoodFence,WoodFenceNE,101,24,17
WoodFence,WoodFenceNE,102,24,14
WoodFence,WoodFenceNE,103,24,19
WoodFence,WoodFenceNE,104,24,20
WoodFence,WoodFenceNE,105,24,21
WoodFence,WoodFenceNE,106,24,22
WoodFence,WoodFenceNE,107,24,23
WoodFence,WoodFenceNW,108,23,26
WoodFence,WoodFenceNW,109,22,26
WoodFence,WoodFenceNW,110,21,26
WoodFence,WoodFenceNW,111,20,26
WoodFence,WoodFenceNW,112,19,26
WoodFence,WoodFenceNW,113,18,26
WoodFence,WoodFenceNW,114,17,26
WoodFence,WoodFenceNW,115,16,26
WoodFence,WoodFenceNE,116,24,24
WoodFence,WoodFenceNE,117,24,25
WoodFence,WoodCornerN,118,24,26
WoodFence,WoodCornerW,119,24,13
WoodFence,WoodFenceNW,120,23,13
WoodFence,WoodFenceNW,121,22,13
WoodFence,WoodFenceNW,122,21,13
WoodFence,WoodFenceNW,123,20,13
WoodFence,WoodFenceNW,124,19,13
WoodFence,WoodFenceNW,125,18,13
WoodFence,WoodFenceNW,126,17,13
WoodFence,WoodFenceNW,127,16,13
WoodFence,WoodFenceNW,128,15,26
WoodFence,WoodFenceNW,129,14,26
WoodFence,WoodFenceNW,130,13,26
WoodFence,WoodFenceNW,131,12,26
WoodFence,WoodFenceNW,132,11,26
WoodFence,WoodFenceNW,133,15,13
WoodFence,WoodFenceNW,134,14,13
WoodFence,WoodFenceNW,135,13,13
WoodFence,WoodFenceNW,136,12,13
WoodFence,WoodFenceNW,137,11,13
WoodFence,WoodCornerS,138,10,13
WoodFence,WoodCornerE,139,10,26
WoodFence,WoodFenceNE,140,10,25
WoodFence,WoodFenceNE,141,10,24
WoodFence,WoodEndSW,142,10,23
WoodFence,WoodFenceNE,143,10,14
WoodFence,WoodFenceNE,144,10,15
WoodFence,WoodEndNE,145,10,16
###Construction###
###Removables###
ClamA,0,0,2,6
ClamB,1,0,2,13
ClamB,2,0,2,18
ClamA,3,0,2,22
ClamA,4,0,2,27
TreeA,5,0,3,13
TreeD,6,0,3,19
ClamB,7,0,4,7
ClamC,8,0,4,15
ClamB,9,0,5,33
ClamB,10,0,6,17
ClamC,11,0,6,20
TreeB,12,0,6,22
TreeA,13,0,7,17
TreeC,14,0,7,27
TreeC,15,0,8,11
ClamA,16,0,8,17
ClamC,17,0,9,13
ClamB,18,0,9,18
ClamB,19,0,9,21
ClamC,20,0,9,26
ClamB,21,0,9,28
TreeD,24,0,10,33
TreeB,25,0,11,4
TreeB,27,0,11,29
TreeB,28,0,12,8
TreeC,30,0,13,30
TreeC,31,0,13,31
TreeD,33,0,14,27
TreeC,34,0,15,2
ClamB,35,0,15,4
TreeC,36,0,15,8
TreeA,38,0,15,33
TreeD,39,0,16,5
TreeC,40,0,17,3
TreeA,41,0,17,11
ClamB,43,0,18,3
TreeC,44,0,18,6
TreeD,45,0,18,8
ClamB,50,0,20,28
TreeC,54,0,23,4
ClamC,55,0,23,11
ClamC,57,0,23,32
TreeD,58,0,24,2
TreeA,59,0,24,9
ClamC,61,0,25,22
ClamC,62,0,26,3
TreeD,63,0,26,9
ClamB,64,0,26,10
ClamB,65,0,26,23
ClamA,66,0,26,24
TreeA,67,0,26,27
ClamB,68,0,26,33
ClamC,69,0,27,6
TreeB,70,0,28,8
TreeB,71,0,28,16
ClamA,72,0,28,17
ClamA,73,0,29,6
TreeD,74,0,29,15
TreeC,75,0,29,18
ClamB,76,0,29,26
TreeC,77,0,29,28
ClamC,78,0,29,31
ClamC,79,0,30,9
ClamC,80,0,30,13
ClamC,81,0,30,15
TreeB,82,0,30,22
ClamA,83,0,30,23
ClamC,84,0,30,27
ClamA,85,0,31,3
ClamA,86,0,31,16
ClamA,87,0,31,23
TreeB,88,0,31,27
TreeD,89,0,31,28
ClamB,90,0,32,10
TreeD,91,0,32,28
ClamA,92,0,32,31
TreeA,93,0,33,3
TreeD,94,0,33,7
TreeA,95,0,33,20
TreeA,96,0,33,23
TreeB,97,0,33,32
###RemovableTimers###
###Numerics###
157,145,160
0.00,0
0,0,0,0,0,0,0,0,0,0
0,0,0,0,0,0,0,0,0,0
0,0,0,0,0,0,0,0,0,0
###Stats###
160,1689,2,0,0,100,92500,96200,471,502500,502500,500,True,True,True,True,True
11/10/2016 9:58:55 PM
###EndofFile###
Additional Campaign Maps
To test campaign, make sure you've added your license code to Map01 scene GameManager > SaveLoadBattle so you can click any of the campaign buttons on the Army menu. Also, we've included a set of 21 campaign files (marked 0000camp00 - 0000camp20) in the download link below.
v7.2 Sample Maps Download Link
https://www.dropbox.com/s/5clocy6v6v5f0f2/v7_demo_map_files.zip?dl=0
How can I make my own campaign maps?
Using the City Building Kit in Unity, design your map. A bit more symmetry - like those pre- arranged maps we see in popular free-to-play strategy games.
The procedure is simple- you run a new game in editor, generate the removables, clean the center, build the walls and then add a few things. It doesn't have to have everything from the start.
Press Local Save to store a copy of the map file on your computer. Retrieve this copy and keep it for further use. Stop the scene and start it again to build another.
Alternatively, you can press Server Save to save a copy online. Just make sure to change the ID in case you accidentally overwrite it when building another map.
There are 21 campaign maps 0000camp00-0000camp20. Campaign maps must use the same filename order as the script loads them by calling these exact filenames (#00-20)
0000camp00.txt | The first campaign (most left button in the screenshot above) |
0000camp01.txt | The second campaign map (located on the bottom of the map) |
0000camp02.txt | The third campaign map (top, next to the first) |
0000camp03.txt | The fourth campaign map |
0000camp04.txt | The fifth campaign map |
0000camp05.txt | The sixth campaign map |
0000camp06.txt | The seventh campaign map |
0000camp07.txt | The eighth campaign map |
0000camp08.txt | The ninth campaign map |
0000camp09.txt | The tenth campaign map |
0000camp10.txt | The eleventh campaign map |
0000camp11.txt | The twelfth campaign map |
0000camp12.txt | The thirteenth campaign map |
0000camp13.txt | The fourteenth campaign map |
0000camp14.txt | The fifteenth campaign map |
0000camp15.txt | The sixteen campaign map |
0000camp16.txt | The seventeenth campaign map |
0000camp17.txt | The eighteenth campaign map |
0000camp18.txt | The nineteenth campaign map |
0000camp19.txt | The twentieth campaign map |
0000camp20.txt | The last campaign map at the furthest right of the scroll screen. |
Can I add more campaigns?
Yes, but you'll have to make changes in a few places. We recommend you first play with the kit and understand how the campaign variables are used (Ctrl+F search through the scripts) before attempting to add additional maps.
To add maps, first you'll need to add additional medallion mission buttons to the ScrollView in the Canvas/ModalsWindows/ArmyWindow/ArmyGroup/ArmyPanel/BottomGroup/ScrollView/Viewport/Content/Waves/Map/Buttons/
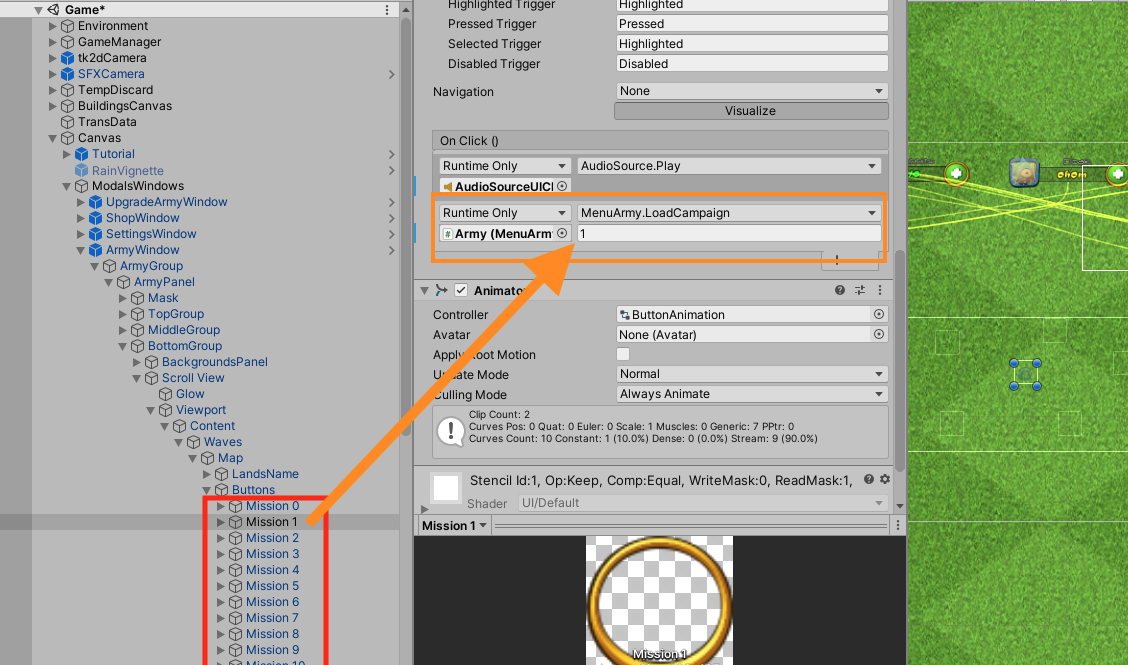
Where the map buttons are located in the hierarchy. Click to view larger.
Each of these medallion buttons is connected to a LoadCampaign() function on tap. Just change the number of the new campaign as seen above.
Plus in the Map01 battle scene you'll need to edit the Campaign No array size with your new elements.
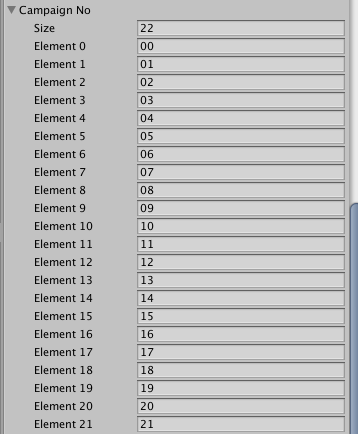
SaveLoadBattle
And lastly, you'll want to make sure you have uploaded to your server new campaign player maps. For example 0000camp21 to 0000camp9999 files. Thes emap files are basically renamed player save files. Not at all difficult to make, just build an enemy base in the Game scene save the file and rename it.
Updated less than a minute ago