FPS Counter
Reminder - Keep Daily Backups!
When working on a strategy game with a kit as big as the Complete Kit - always keep a working daily backup! Save yourself the trouble of rolling-back changes and losing work.
What is the FPS Counter?
For developers, the City Building Kit includes the Unity FPS counter for visual tracking of the performance of your game located in Scripts/Camera/FPSCounter.js
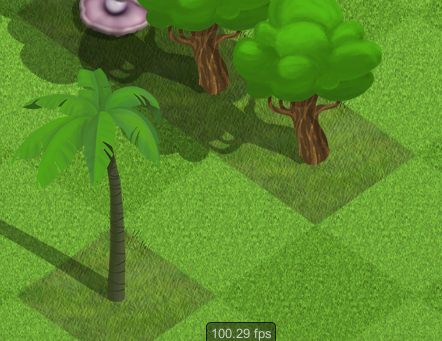
Click to view larger.
Usually on our development machines we get 30-100 FPS depending on the gameplay (older Macbook Pro 2010 models)
Code reference
Open Scripts/Camera/FPSCounter.js and you'll see the following FPS counter provided by Unity.
var updateInterval = 1.0;
private var accum = 0.0; // FPS accumulated over the interval
private var frames = 0; // Frames drawn over the interval
private var timeleft : float; // Left time for current interval
private var fps = 15.0; // Current FPS
private var lastSample : double;
private var gotIntervals = 0;
function Start()
{
timeleft = updateInterval;
lastSample = Time.realtimeSinceStartup;
}
function GetFPS() : float { return fps; }
function HasFPS() : boolean { return gotIntervals > 2; }
function Update()
{
++frames;
var newSample = Time.realtimeSinceStartup;
var deltaTime = newSample - lastSample;
lastSample = newSample;
timeleft -= deltaTime;
accum += 1.0 / deltaTime;
// Interval ended - update GUI text and start new interval
if( timeleft <= 0.0 )
{
// display two fractional digits (f2 format)
fps = accum/frames;
// guiText.text = fps.ToString("f2");
timeleft = updateInterval;
accum = 0.0;
frames = 0;
++gotIntervals;
}
}
// Displays the FPS in Unity
function OnGUI()
{
GUI.Box(new Rect(Screen.width/2 -35, Screen.height -20, 70, 25), fps.ToString("f2") + " fps");// | QSetting: " + QualitySettings.currentLevel
}
You'll notice at the end of the script the function OnGUI places the FPS string value calculated on the screen in a simple grey GUI box.
// Displays the FPS in Unity
function OnGUI()
{
GUI.Box(new Rect(Screen.width/2 -35, Screen.height -20, 70, 25), fps.ToString("f2") + " fps");// | QSetting: " + QualitySettings.currentLevel
}
How to disable the FPS GUI
For production games, you don't need to display this FPS GUI - so either comment out the contents of the Scripts/Camera/FPSCounter.js script or comment out the GUI.Box in the OnGUI function like the excerpt below:
// Displays the FPS in Unity
function OnGUI()
{
//GUI.Box(new Rect(Screen.width/2 -35, Screen.height -20, 70, 25), fps.ToString("f2") + " fps");
}
Updated less than a minute ago